Hi! I`m Chan. It`s the first post of my blog. In this post, I`ll talk about SkeletonStream of Kinect SDK based on C. As you know that, there are lots of examples of Kinect programming based on C#, but not on C.
In this programming, I used OpenCV Library v2.4.2 and Kinect SDK v1.6 on 64bit System.
Kinect SDK supported the development in C/C#/VB. Nowadays, MS offers lots of examples in C#/VB, because c#/VB works on .net framework made by MS.
But there are limitations of C#. In Computer Vision parts, OpenCV Library and PointCloud Library is the most powerful tools of Image processing. But OpenCV & PCL can be only developed in C / Python. It cannot worked on c#. Of course, There is alternative way to process image in C#, in example EmguCV, OpenCVSharp. etc..
So that`s why I Program Kinect SDK in C. In short, I can use OpenCV or PCL.. and Maybe DirectX.
Let`s start! Open the new empty project in VC++ named Color.(Because I`ll announce about ColorStream Programming next post)
At First, We need to decide which mode I work on this application : 32bit or 64bit and debug mode or release mode...
Because without this, We`ll have a problem to correct all of this settings. So Keep in mind to select development system at first. I`ll assumed the environment : Release & 32bit.
Click the Project name in Project Solution with right mouse button. and select the properties. As you can see. There are lots of settings in this properties. The Things we need to modify are VC++ Directories and Linker.
First of all. select the VC++ Directories and modify the Include Directories like under below:
In My case, OpenCVRoot is my environment variable of installed OpenCV directories. If you don`t know about it, just select the folder button and choose the path of OpenCV. In this process, We include header files in our projects.
Next, we need to modify the libraries Directories. Modify the folder path like under below:
As I mentioned it before, It`s important to select the mode. That`s because, there is difference of OpenCV Libraries between Debug and release.
Finally, Select the Linker part and choose the Input. you can see the blank Additional Dependencies. we need to insert the library files in this blank. put the file name in this blank like this:
If you have a plan to develop in debug mode, The library file name must include 'd' character. like opencv_highgui242d.lib You can find it in your OpenCV Directories/lib
and Finally we need to insert Kinect SDK Library in here.
That`s it. If you want to know how I modify this properties correctly, Just insert the header file like this:
If you set correctly, the intelisense of Visual C++ will find the exact header files of OpenCV, and so will Kinect header file. If you want to use the feature of Kinect, just put the NuiApi.h in your project.
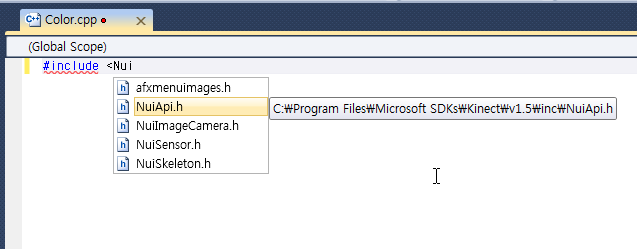
There are 4 header files related in Kinect SDK. but the only thing I need to include is NuiApi.h.
That`s all. I tried to make a simple app of showing Color and Depth Image using OpenCV
If you have a question of setting Kinect SDK in C, please feel free to ask :)
* You can read Korean or you can use language translator, You`ll find more details on my main blog :
http://talkingaboutme.tistory.com/221